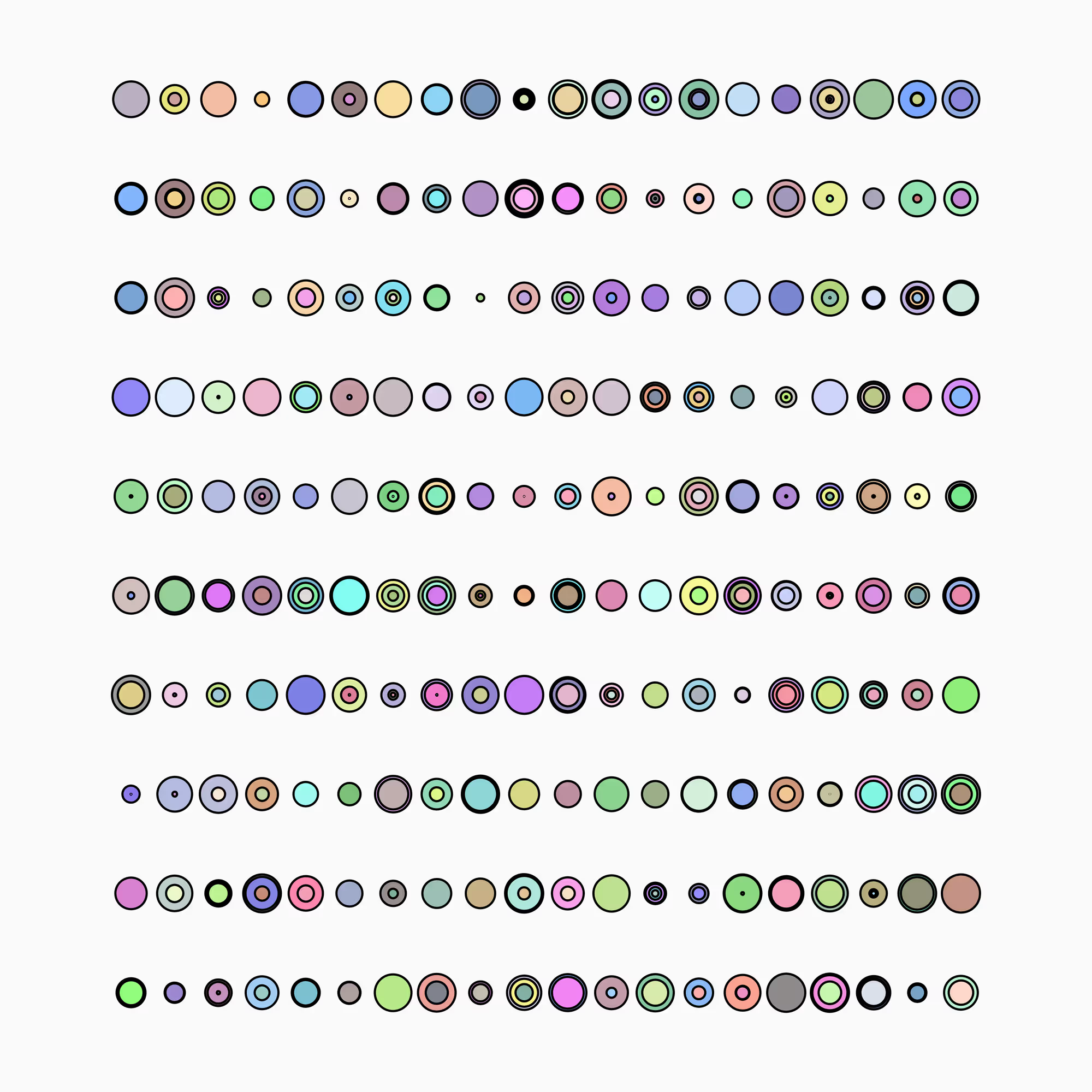
I attended a Processing Jam this week!
Processing is a flexible software sketchbook and a language for learning how to code. Since 2001, Processing has promoted software literacy within the visual arts and visual literacy within technology. There are tens of thousands of students, artists, designers, researchers, and hobbyists who use Processing for learning and prototyping.
I haven’t used Processing since around 2020, when I created a few sketches as an homage to an exhibit I saw at the AGO of Margaret Priest’s construction series. I’ve long been inspired by people like Tyler Hobbs and Matt Deslauriers who are making some pretty wild, cool stuff with code.
It was fun to crack open Processing again — in my case, I use a wrapper around Processing in Clojure called quil. After looking up quil again, other than a few threads that asked "is quil still being maintained," etc., I got it working with no problems.
I started by drawing a few ideas in my sketchbook to see what I could come up with. Then I hacked away at rendering a bunch of circles on the screen. Here’s how it came out:
Most of this came as a result of mashing code until it came together. I had some help from a friend at the gathering to center everything (I always trip over centering things in Processing).
I’d like to do more creative coding, but it’ll have to get in line behind the game I’m building (which is sufficiently creative-coding already).
Find the code below! It was fun to write Clojure again; I kind of forgot how expressive it is.
(ns mar-20.core
(:require [quil.core :as q]
[quil.middleware :as m]))
(def w 4500)
(def h 4500)
(defn setup []
(q/frame-rate 30)
{:angle 0})
(defn update-state [state]
; Update sketch state by changing circle colour and position.
{:angle (+ (:angle state) 1.1)})
(defn rnd-col []
(q/fill
(q/random 120 255) (q/random 120 255) (q/random 120 255)))
(defn draw-state [state]
(let [margin-pc 0.1
width-for-circles (* w (- 1 (* 2 margin-pc)))
margin (* w margin-pc)
num-circ 20
circle-max-size (/ width-for-circles num-circ)
circle-shrink-px (* circle-max-size 0.1)
num-rows 10]
(q/smooth)
(q/no-loop)
(q/background 250)
(doseq [hloop (range num-rows)
:let [h-offset (/ h (inc num-rows))
circ-y (+ (* (+ hloop 1) h-offset))]]
(doseq [iloop (range 4)]
(doseq [jloop (range num-circ)
:let [
circle-size (- circle-max-size (q/random 3 (- circle-max-size (/ circle-max-size 10))) circle-shrink-px)
circle-diff (- circle-max-size circle-size)
circ-x (+ (* jloop circle-max-size) margin (/ circle-max-size 2))
]
]
(q/stroke-weight (* w 0.002))
(rnd-col)
(q/ellipse circ-x circ-y circle-size circle-size)))))
(q/save "test.png"))
(q/defsketch mar-20
:title ""
:size [w h]
:setup setup
:update update-state
:draw draw-state
:features [:keep-on-top]
:middleware [m/fun-mode])
❦